How to Avoid Dependency Conflicts in Android Multi-Module Apps
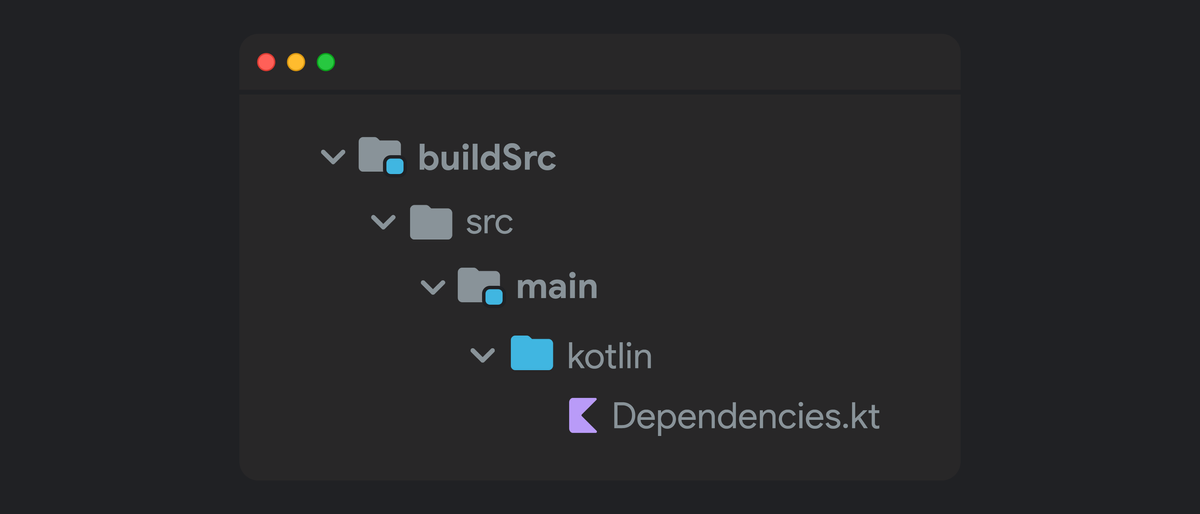
As an Android developer, you may have experienced the frustration of managing dependencies in your project. It can be especially challenging when you have multiple modules in your project, as it's easy to end up with different versions of the same dependency in different modules. This can lead to conflicts and unpredictable behavior.
One way to solve this problem is to create a separate buildSrc
module in your project. The buildSrc
module is a special module that is used to store your project's build logic, including dependencies. By keeping your dependencies in one place, you can ensure that all modules in your project are using the same version of a dependency.
There are of course different ways of solving this problem, and which approach is best for you will depend on your specific needs and preferences.
When I posted this blog post, I figured that most people these days prefer using Gradle version catalog. I personally find buildSrc
easy to maintain.
So..
To create a buildSrc
module, you'll need to do the following:
- Open your project.
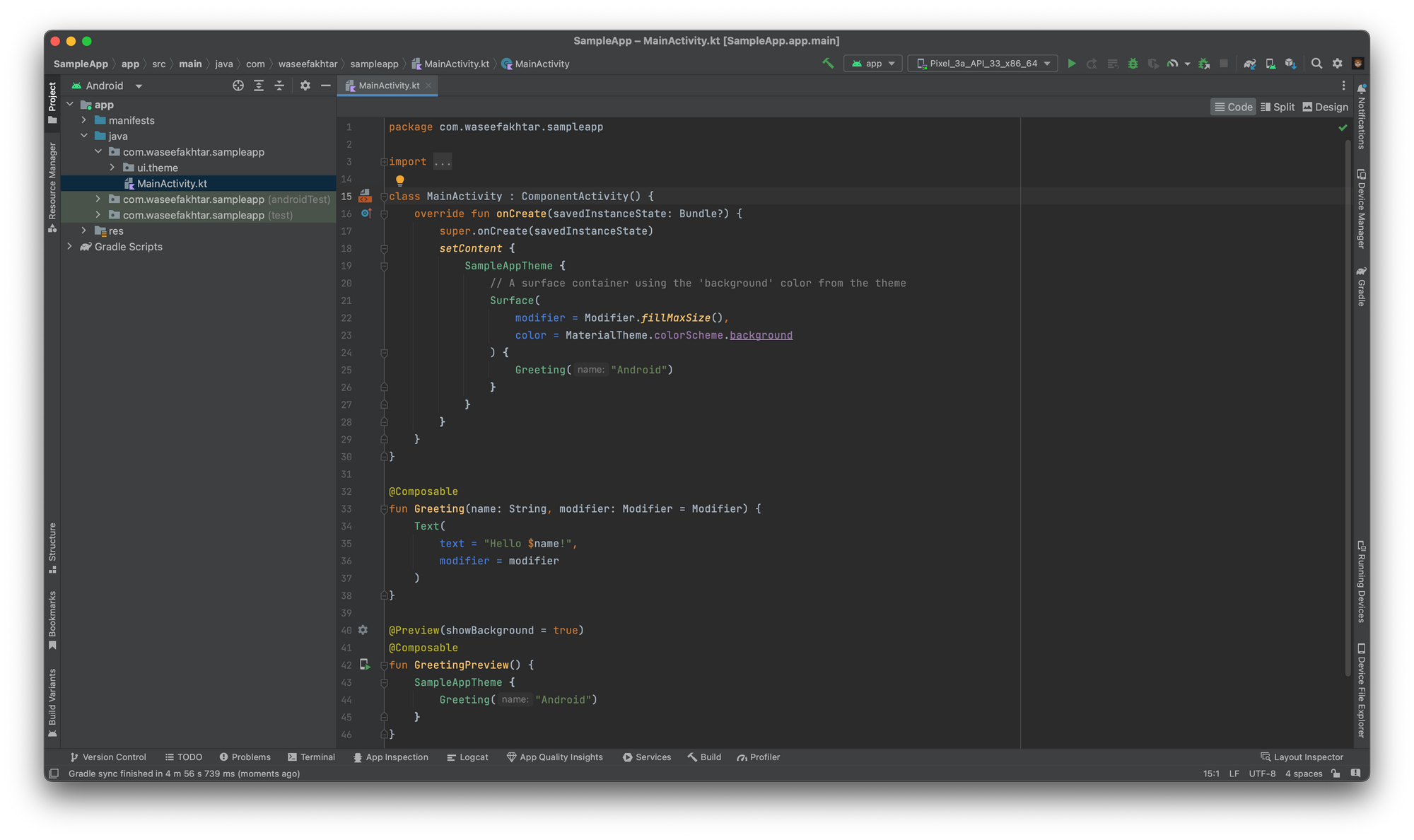
2. From the Project pane, change viewing your files from Android to Project Files.
When you view the files as Android files, they are organized according to their function within the Android operating system, such as by activity or service. When you view the files as Project files, they are organized according to their location in the file system.
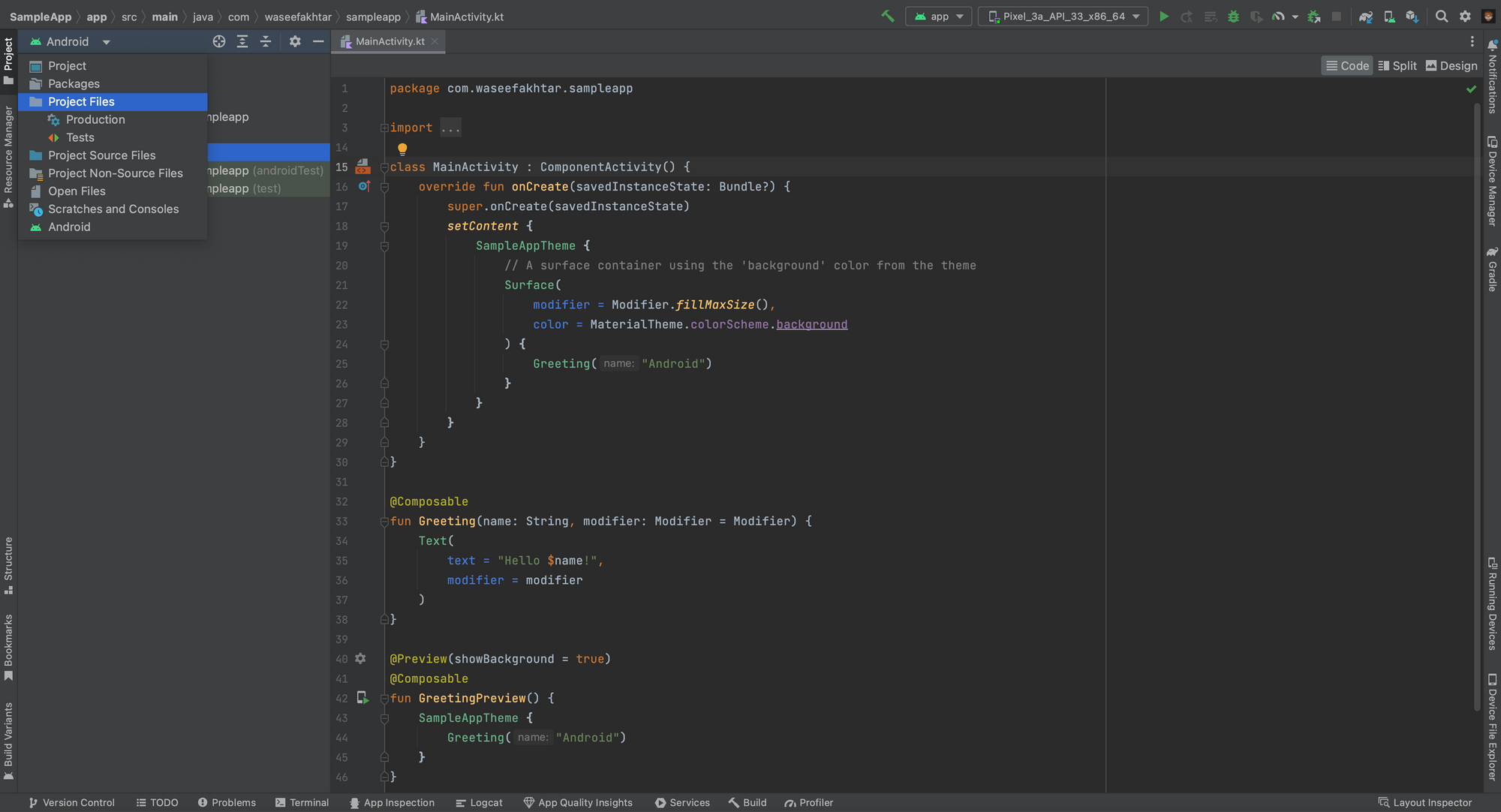
Here's an example of how the files would show when you view the files as Project files – they are organized according to their location in the file system. 👇
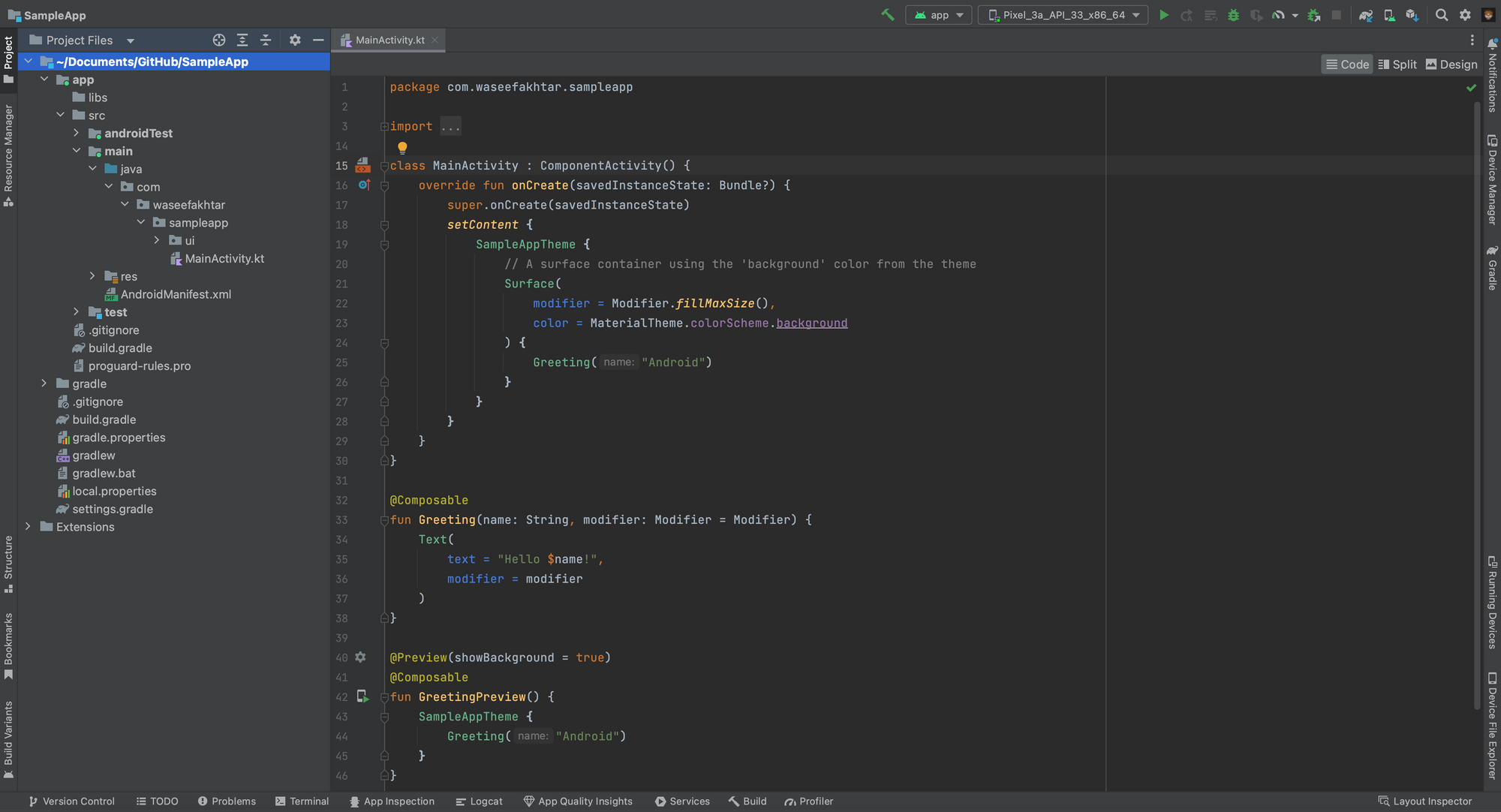
3. Now, create a new directory from your project root folder, And name it buildSrc
.
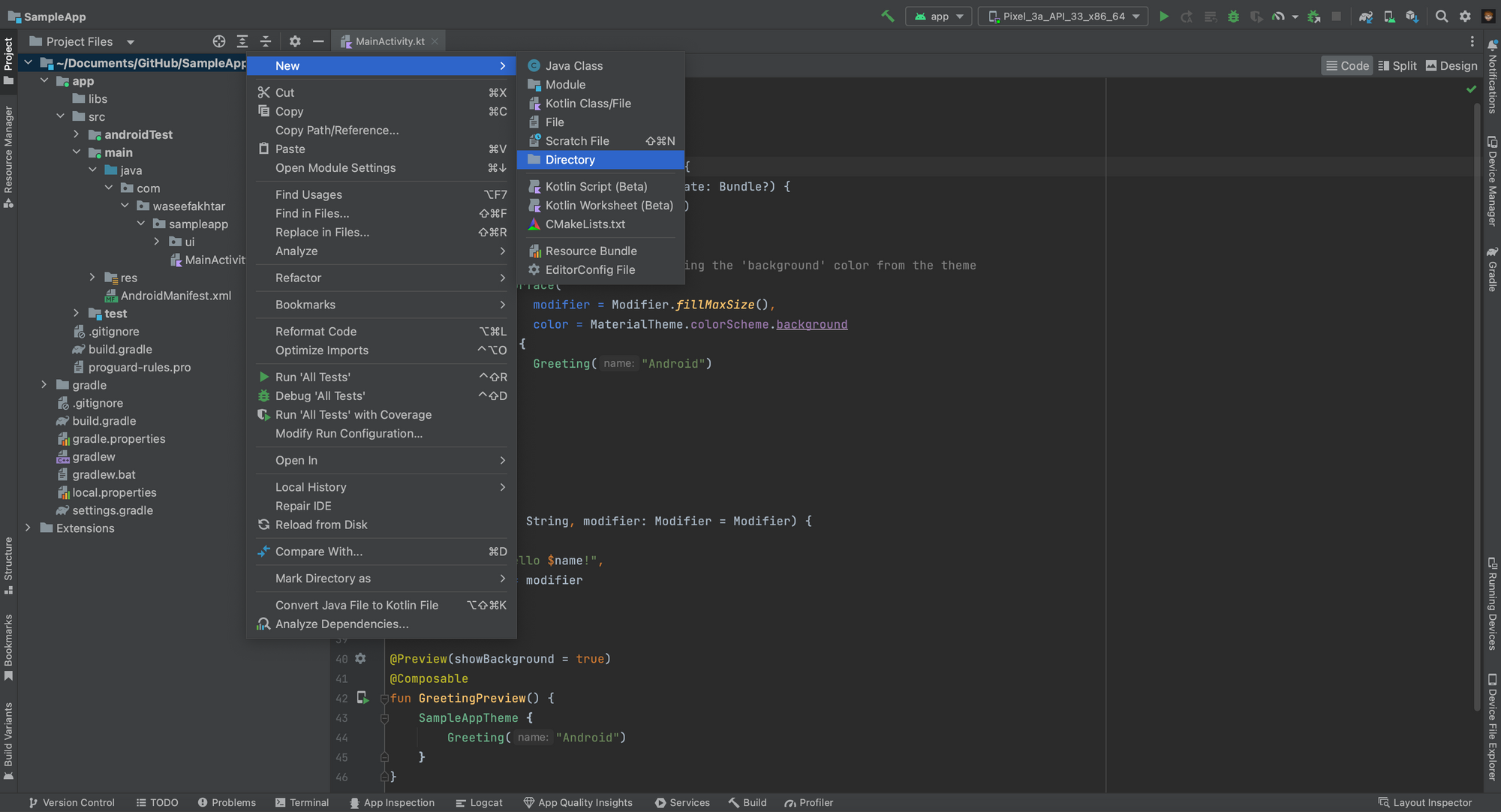
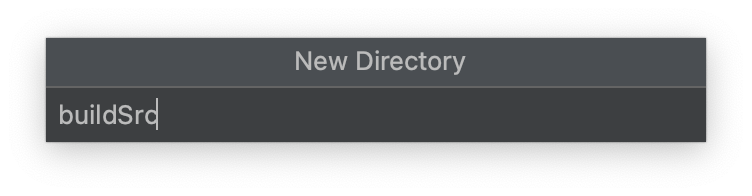
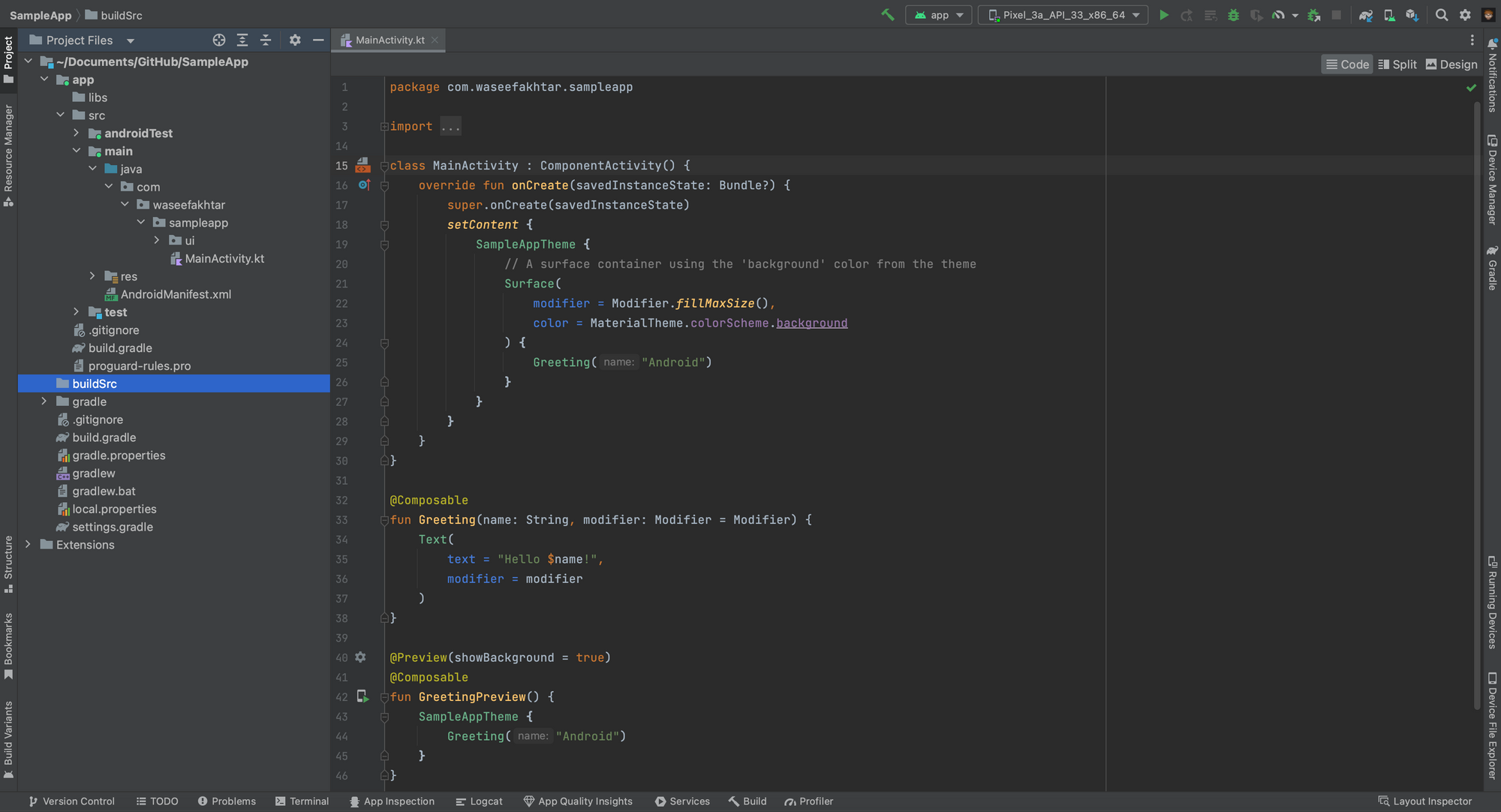
4. Now we need to create a new gradle file inside this directory.
This will help turn this from a simple directory to a module. This will also then help compile the dependencies from this module into our other gradle files in different modules.
By turning a directory into a module, you can create a separate unit of code within your project that can be compiled and run independently. This can be useful for organizing your project and for creating reusable code that can be shared across multiple apps or libraries.
Here's how you do it:
a) Create a new file and name it build.gradle.kts
.
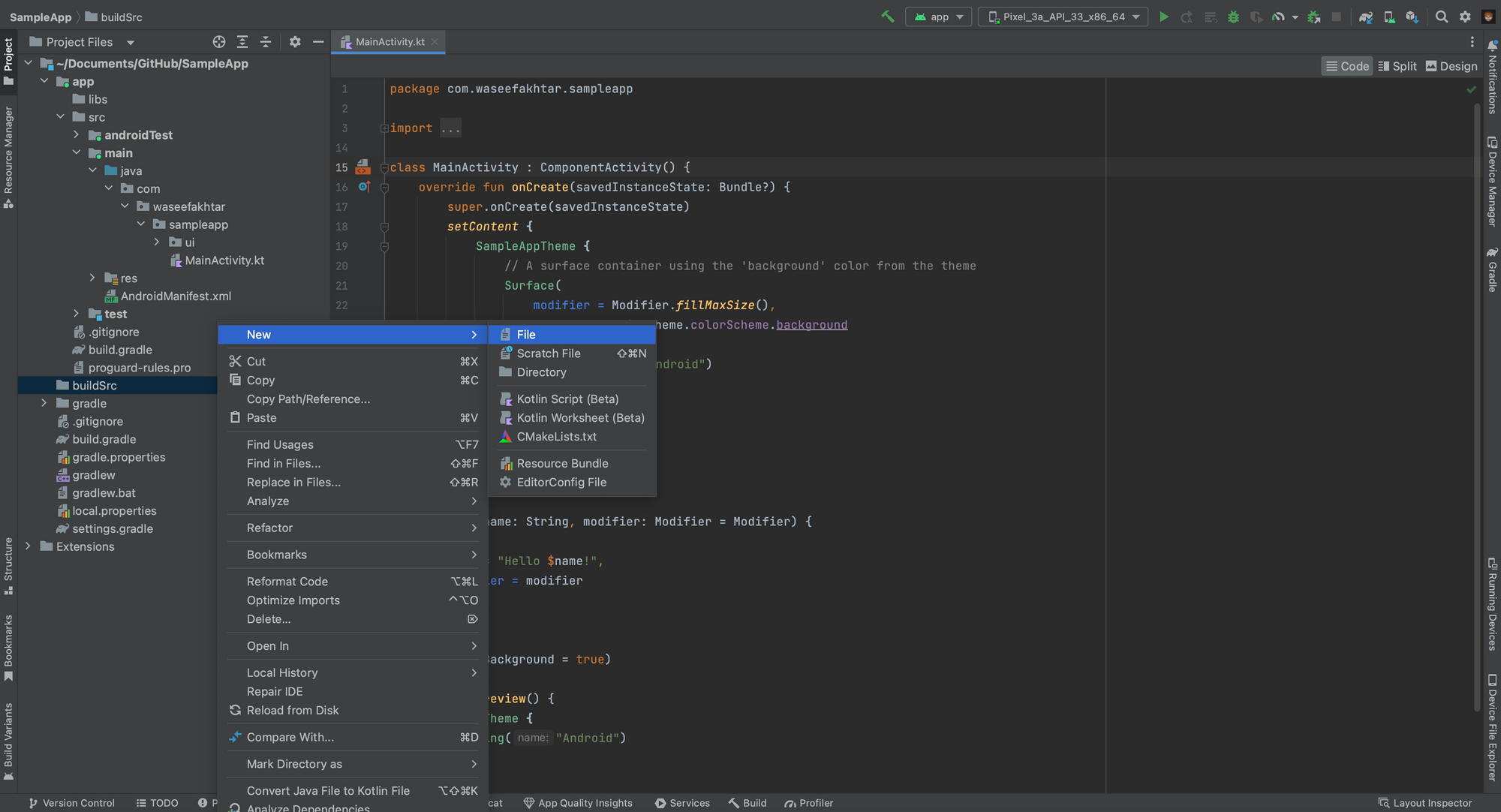
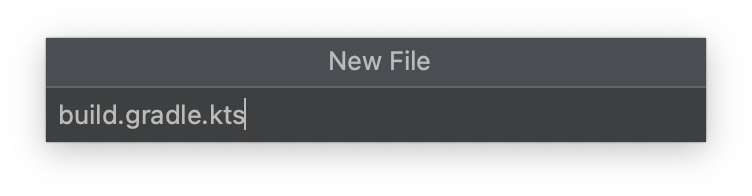
b) Paste the following code in your new file:
c) Sync the project to make the new changes work across the project.
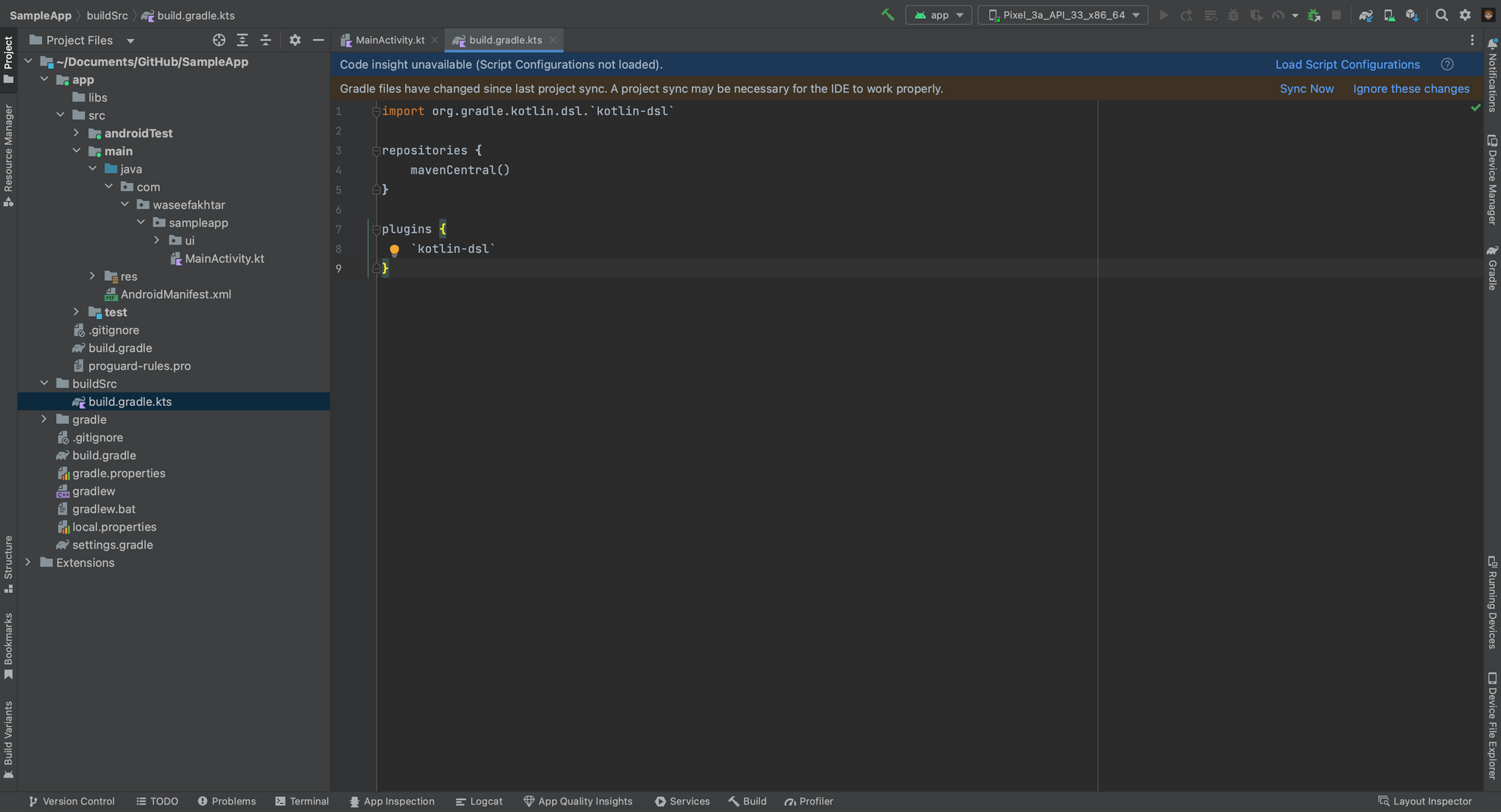
If you notice, this adds a little blue squared icon at the bottom right of your buildSrc
folder icon and also add build directory inside it.
This means the sync was successful in turning the directory into a module.
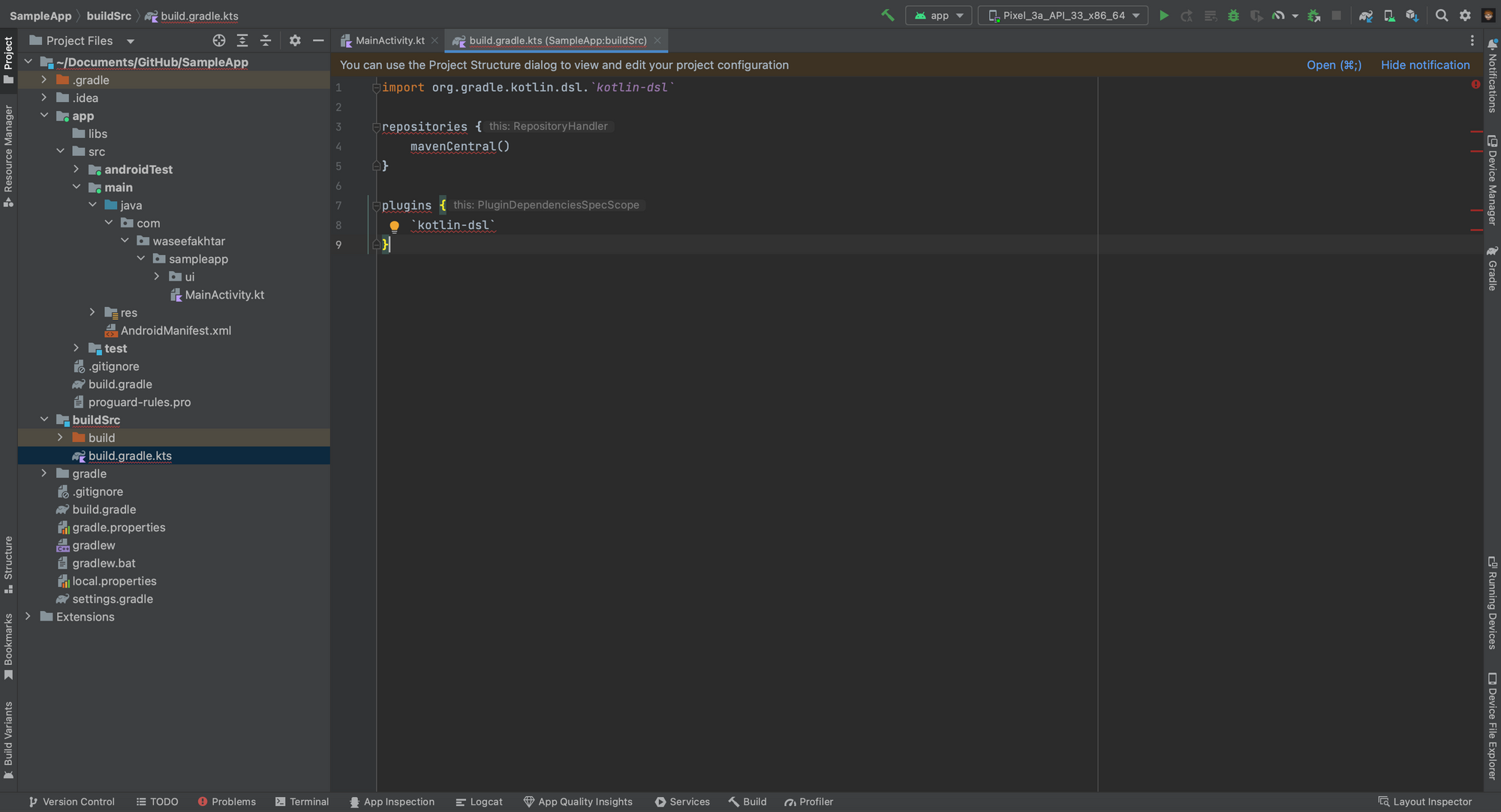
5. Time to add dependencies!
a) In your buildSrc
, create a new src/main/kotlin
directory.
This is used to store all your Kotlin source code for this module – including how we will declare dependencies.
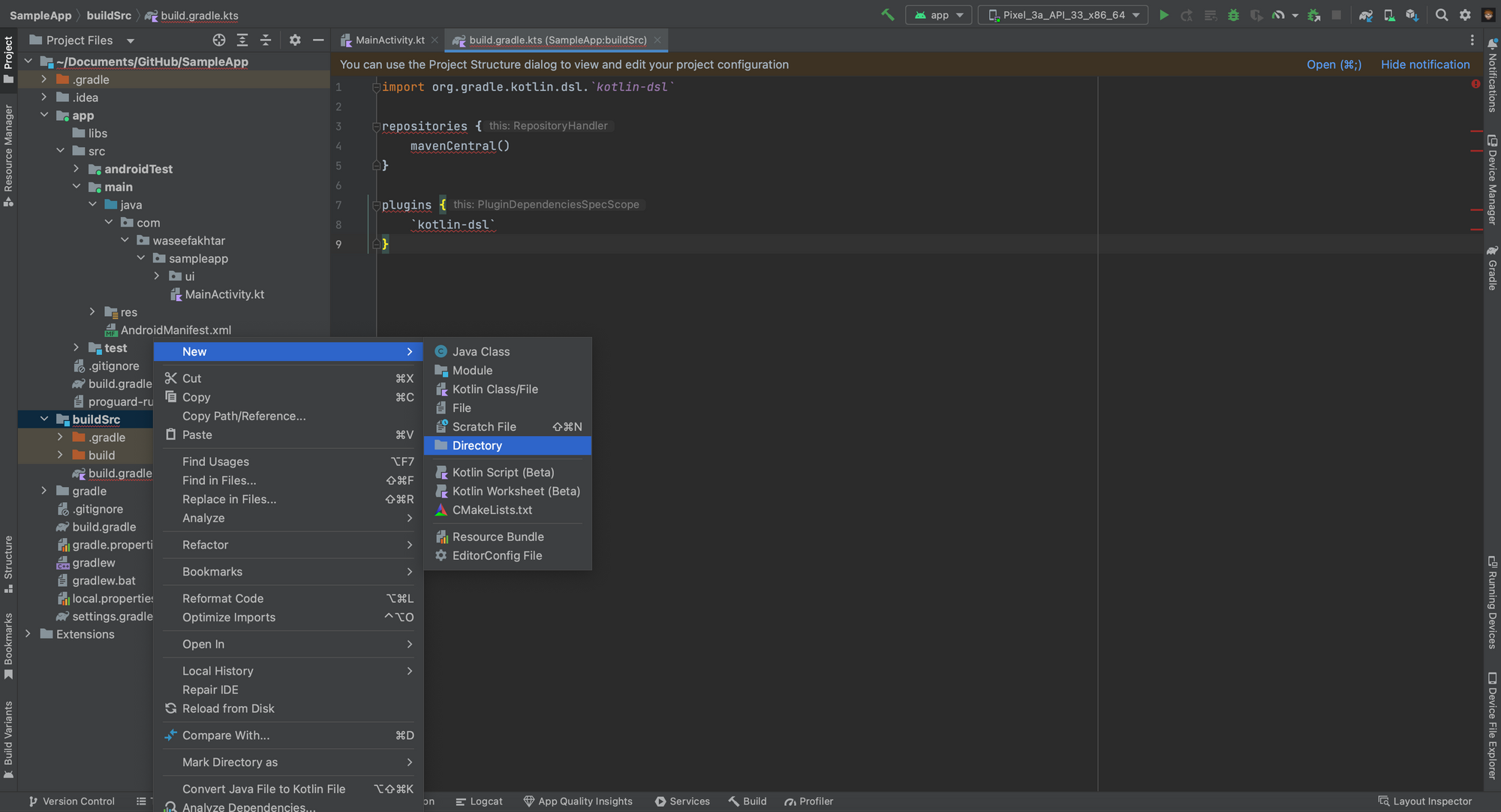
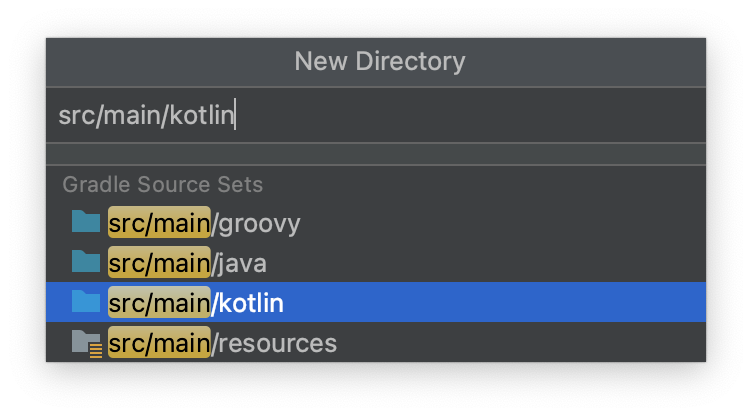
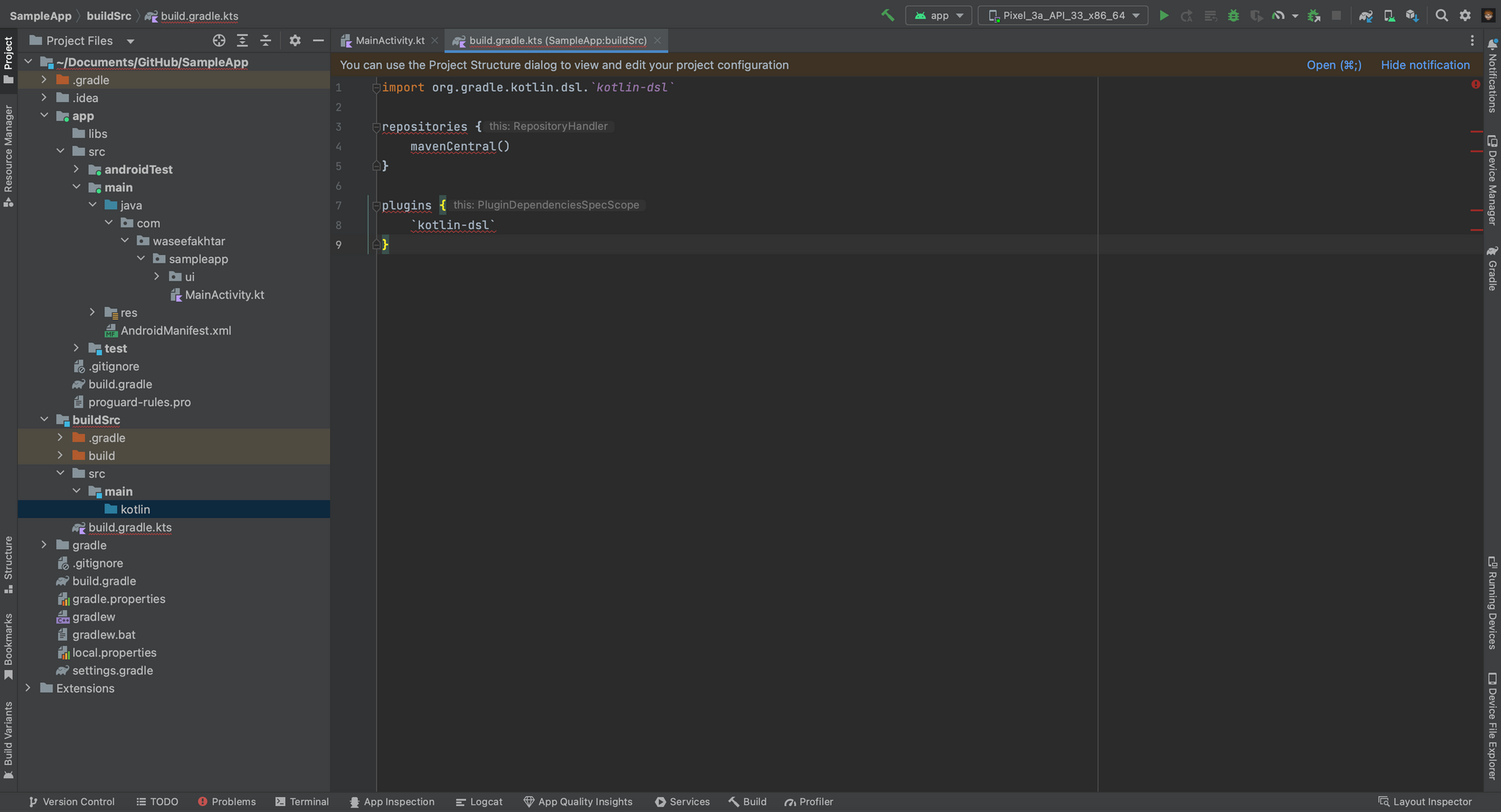
6. Finally, inside the src/main/kotlin
directory, create a new Kotlin object class named, Deps
, or Dependencies
.
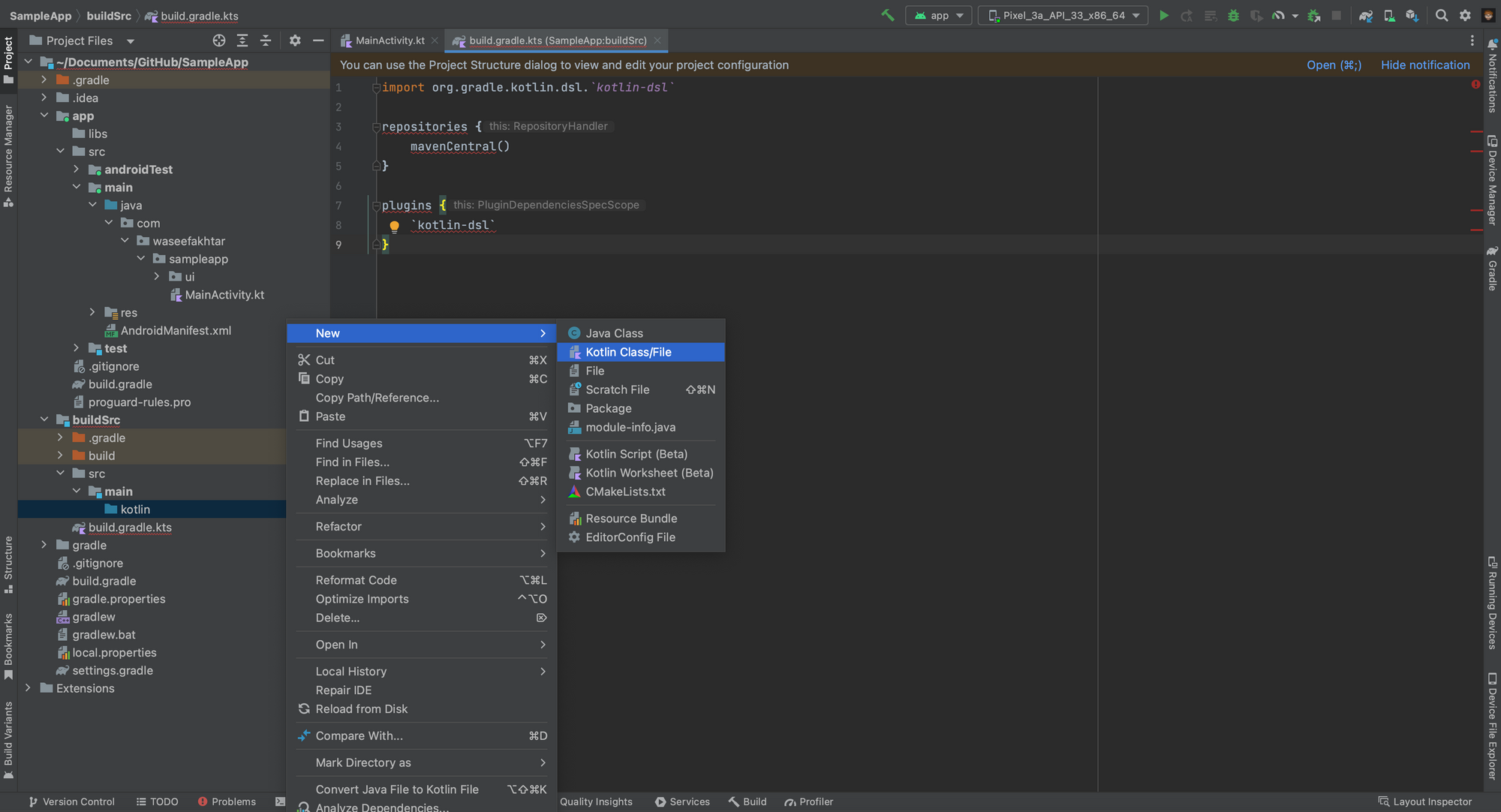
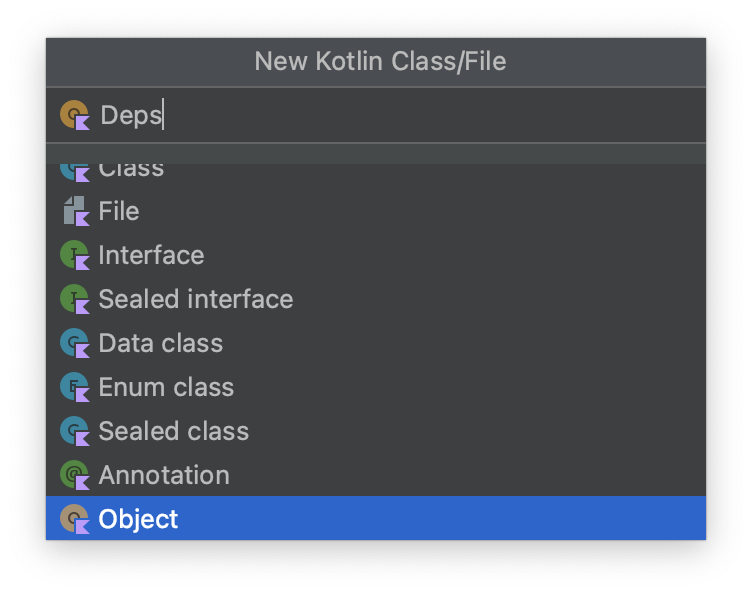
7. Paste the dependencies you'd like for your project.
Make it so that the dependency version has its own variable. This ensures that we use the same version for all the similar dependencies. For instance, compose-ui, compose-ui-tooling, compose-foundation, etc all use the same version so you won't run into conflicts or errors by using different versions for different dependencies.
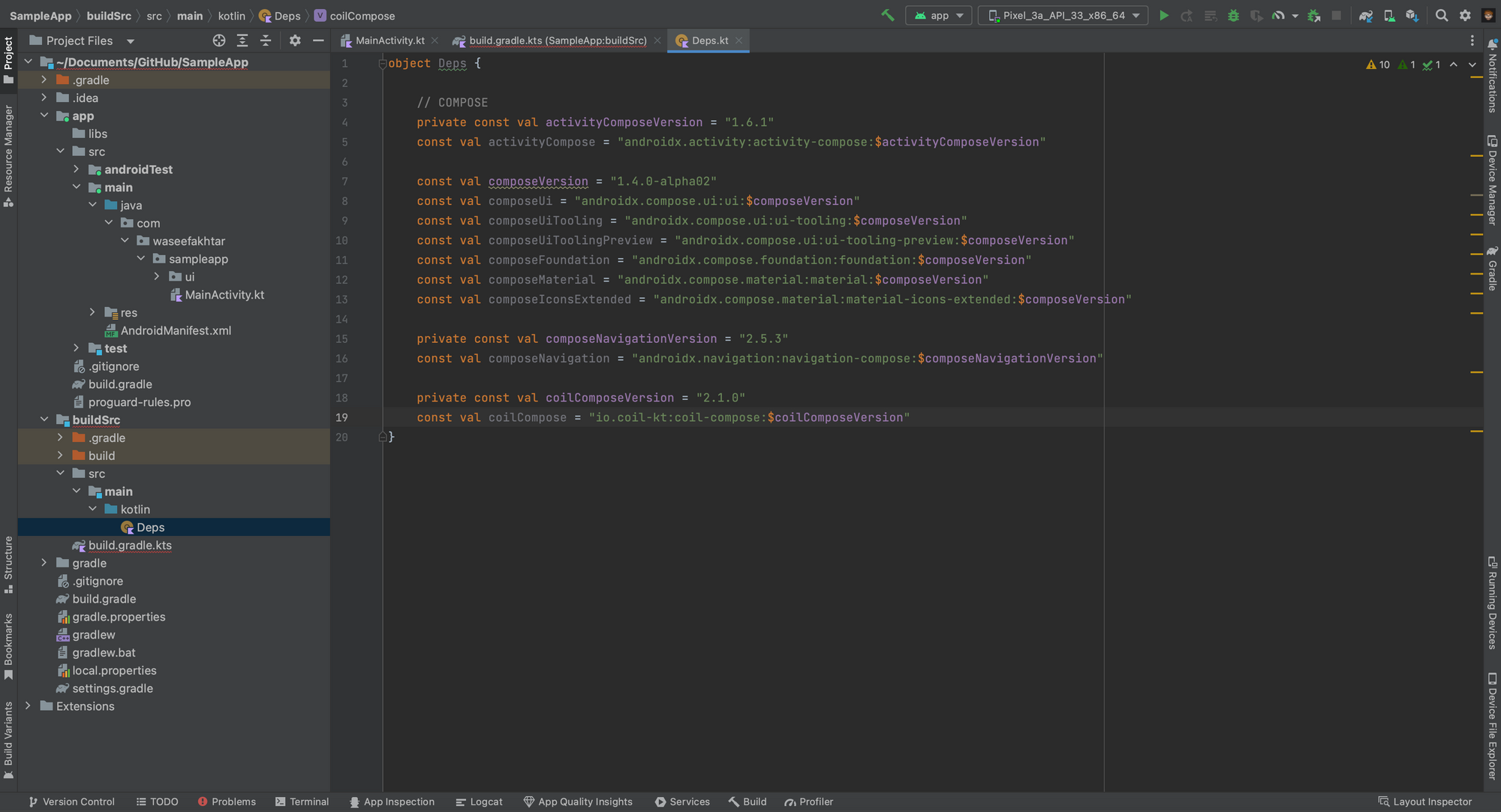
8. Now all you need is to go to other modules' gradle file, and replace your already defined dependencies with the ones we defined in the Deps.kt
object class.
For instance:
implementation 'androidx.compose.ui:ui'
can be replaced by implementation(Deps.composeUi)
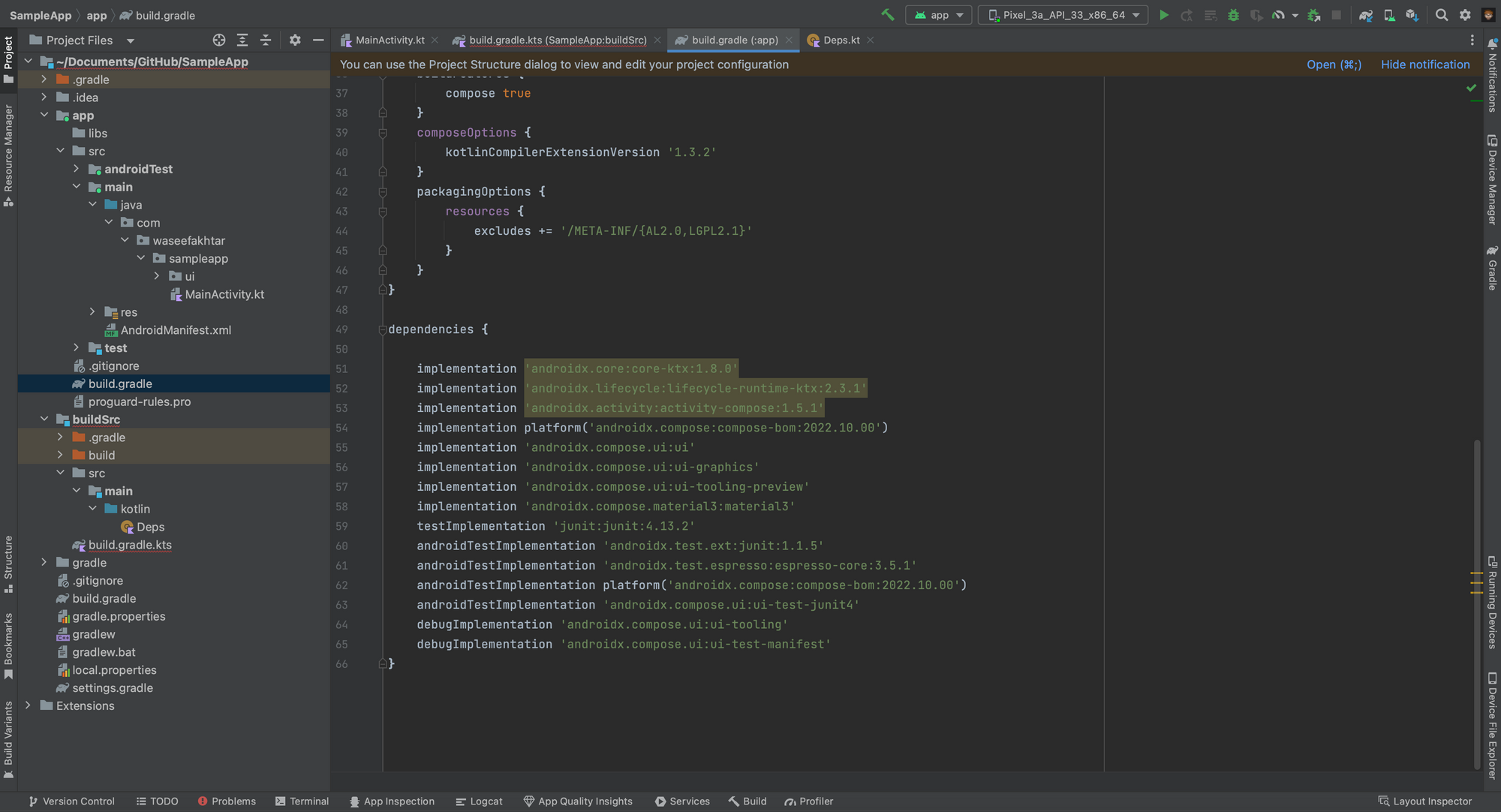
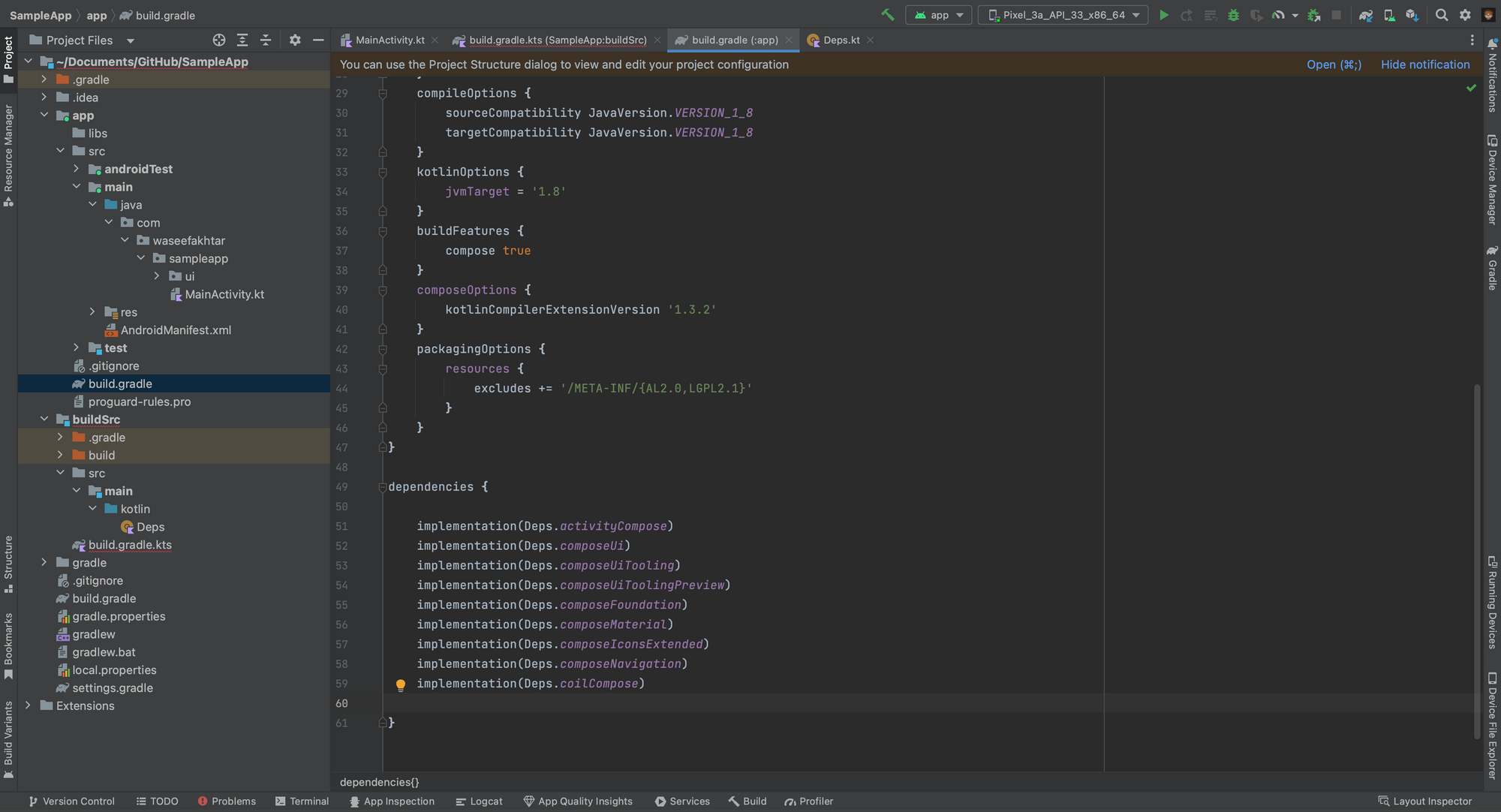
And.. congratulations! You now have a more scalable, structured, and maintainable new project for your Android app.
--
By keeping your dependencies in the buildSrc
module, you can avoid the risk of keeping different versions of dependencies in different modules. This can make it easier to manage your project and ensure that everything is working as intended. Plus, it can make it easier to refactor your code and make changes, as you'll only need to update the dependencies in one place.
Overall, creating a buildSrc
module is a simple and effective way to manage dependencies in your Android project. It can save you time and hassle in the long run, and help you create a more stable and reliable app.
Awesome that you came this far! 👏 Now I'd love to know what the most annoying part of this post was or if it was of any help to you. Either ways, you can drop me a DM on: www.twitter.com/waseefakhtar ✌️
Also, if you're new to Android development, or have heard a lot abt this new thing called Jetpack Compose, I just have the right blog post to get you started:
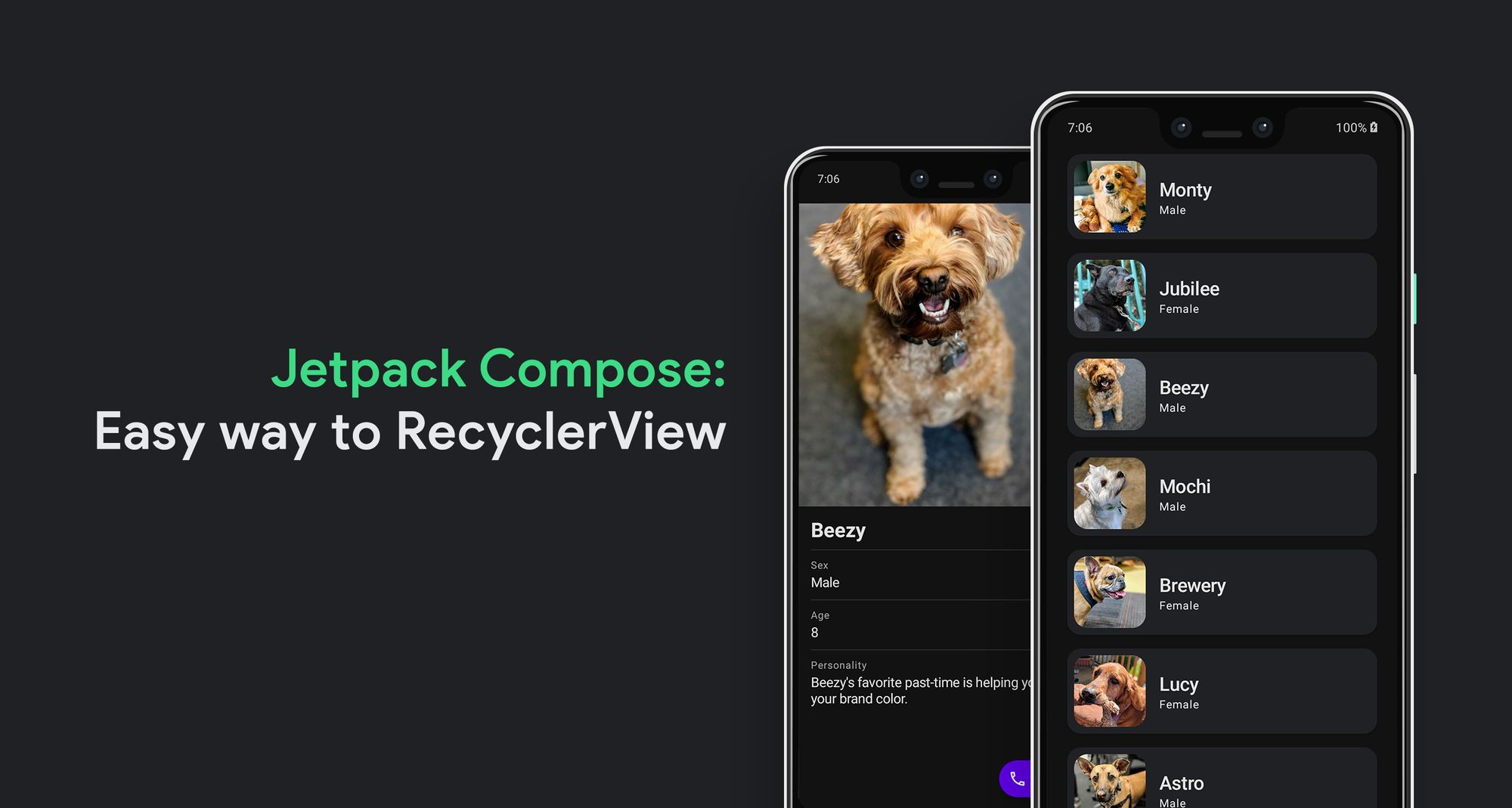
Happy Coding! 💻